Introduction to Acrobat Sign API
More than 50,000 enterprises, including Tesla, Microsoft, Hitachi, and HSBC, use Adobe Acrobat eSign. It helps speed up transactions by 30% and has saved $8.7 million in sustainability costs. Users and reviewers consistently rank it as a top choice for secure and reliable electronic signatures.
What Is Adobe Acrobat Sign?
Adobe Acrobat is a cloud-based solution that provides eSignature services. It helps you create,
track, and sign eSignatures. You can also accept digital payments and securely store
documents.
Why Integrate Adobe Acrobat Sign via API?
Integrating Adobe Acrobat Sign via API allows developers to automate document-related tasks, reducing manual intervention. It enables seamless document workflows, comprehensive document management, real-time tracking, and advanced features like bulk document processing and webhook integrations. This setup streamlines tasks and boosts efficiency by organizing documents effectively and allowing for quick monitoring and automated updates. With the added benefit of Acrobat AI Assistant, you can efficiently analyze multiple documents, summarize information, and outline key points to take smarter actions.
Getting Started With Adobe Acrobat Sign API: Authentication and Setup
Setting Up Your Adobe Acrobat Sign Account
To get started with Adobe Acrobat Sign account:
- Create an Acrobat Sign Developer account: Go to the Adobe Acrobat Sign website and sign in with your business information.
- Set user roles and permissions: First, navigate to account settings. In the ‘Users’ section, you can add or manage users. You can assign them roles such as admin, user, and group admin on their access needs. Admin has full control over the account settings and integrations. Users can send documents for signatures. Group Admin can manage specific user groups within the account.
- Configuring Account Settings: In the account settings go to the Branding section. Set up default branding, email templates, and authentication settings. Under the Compliance section, review and customize e-signature workflows and data retention policies.
API Access and Authentication
To access the Adobe Acrobat Sign API, you need to generate API keys and tokens and create an OAuth 2.0 flow which enables secure communication with the Adobe Sign servers.
- Generating API keys and tokens
- Create a new project under My Projects. Select your project and navigate to APIs.
- Click on Add API and choose Adobe Acrobat Sign API.
- Under the Credentials tab, click Generate API Key (Client ID) and Generate Client Secret.
- Overview of OAuth 2.0 for Adobe Acrobat Sign
- To initiate the OAuth 2.0 authorization flow, use the Client ID, Client Secret, and Redirect URI (set in the project).
- Direct users to Adobe’s authorization URL
- After the user grants permissions, Adobe redirects to your Redirect URI with an authorization code.
- When you make a post request, you provide an access token in exchange for an authorization code.
- Retrieve the access token from the response to use it for subsequent API requests.
- API Rate Limits and Errors
- Rate Limits: API request limits vary by account level (per minute/hour). Adobe Acrobat limits transactions based on the service level of the sending party.
- Error 429 (Too Many Requests): This error occurs when a server detects that a client has sent too many requests in a given amount of time.
Understanding Adobe Acrobat Sign API Endpoints
Key API Endpoints
Acrobat Sign REST APIs can integrate signing functionalities into your application. Here are the most commonly used API Endpoints:
Also see: Adobe Acrobat Sign API Directory
Agreements API
- Purpose: Manages document workflows including sending, tracking, and managing agreements.
- Common Use Cases: Sending documents for signatures, retrieving the status of agreements, and tracking completion.
- Example: For creating an agreement, send a POST request to the /agreements endpoint:
POST /api/rest/v6/agreements HTTP/1.1
Host: api.na1.echosign.com
Authorization: Bearer 3AAABLblNOTREALTOKENLDaV
Content-Type: application/json
{
"fileInfos": [{
"transientDocumentId": "<copy-transient-from-the-upload-document-step>"
}],
"name": "MyTestAgreement",
"participantSetsInfo": [{
"memberInfos": [{
"email": "signer@somecompany.com"
}],
"order": 1,
"role": "SIGNER"
}],
"signatureType": "ESIGN",
"state": "IN_PROCESS"
}
User API
- Purpose: Manages user accounts, roles, and permissions.
- Common Use Cases: Creating, retrieving, updating, or deleting user accounts.
Workflow API
- Purpose: Automates document processes and workflows.
- Common Use Cases: Creating and managing document workflows and automated processes.
API Request and Response Structure
Adobe Acrobat Sign API allows you to manage agreements, users, and workflows using common HTTP methods:
- GET: Retrieve data (e.g., agreements, users).
- POST: Send data to create new resources (e.g., send agreements).
- PUT: Update existing resources (e.g., modify user info).
- DELETE: Remove resources (e.g., delete workflows).
Example: Creating a User (POST Request)
To create a user using the Adobe Acrobat Sign API, provide the required parameters such as authorization and DetailedUserInfo. Structure your request in JSON format, specifying key-value pairs.
Sample JSON
{
"email": "newuser@example.com",
"firstName": "Augustus",
"lastName": "Green",
"company": "ExampleCorp"
}
Sample Python Code
import requests
# Replace with the correct URL for the Create User API
url = https://api.na1.adobesign.com/api/rest/v6/users
# API headers including your OAuth Bearer token
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN", # Replace with your valid access token
"Content-Type": "application/json"
}
# User details (Modify these details as needed)
data = {
"email": "newuser@example.com", # The email of the user you want to create
"firstName": "John", # First name of the user
"lastName": "Doe", # Last name of the user
"company": "ExampleCorp" # The company the user belongs to (optional)
}
# Sending the POST request to create the user
response = requests.post(url, json=data, headers=headers)
# Output the response from the API (response will be in JSON format)
print(response.json())
Key Response Fields
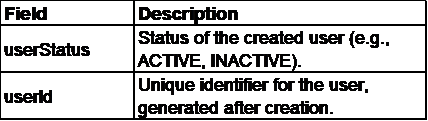
Basic API Integration Steps
Making Your First API Call
To authenticate your application, you'll need an OAuth 2.0 access token. Section 2.2: API Access and Authentication explains how to generate API keys and tokens. Once you have your access token, you’re ready to make your first API call.
Retrieving the status of an agreement using the GET method. This is a common use case for checking the progress of a document sent for signature.
Step-by-Step Process of a Basic API Call
- Sending an Agreement: The agreement’s API section explains the process of sending an agreement.
- Retrieving Agreement Status: To retrieve the agreement status, make a GET request to the /agreements/{agreementId} endpoint.
Set the endpoint and send the GET request:
GET /api/rest/v6/agreements/3AAABLblqZNOTREALAGREEMENTID5_BjiH HTTP/1.1
Host: api.na1.echosign.com
Authorization: Bearer 3AAANOTREALTOKENMS-4ATH
{
"id": "<an-adobe-sign-generated-id>",
"name": "MyTestAgreement",
"participantSetsInfo": [{
"memberInfos": [{
"email": "signer@somecompany.com",
"securityOption": {
"authenticationMethod": "NONE"
}
}],
"role": "SIGNER",
"order": 1
}],
"senderEmail": "sender@somecompany.com",
"createdDate": "2018-07-23T08:13:16Z",
"signatureType": "ESIGN",
"locale": "en_US",
"status": "OUT_FOR_SIGNATURE",
"documentVisibilityEnabled": false
}
- Managing Agreements: You can also manage existing agreements by using the PUT and DELETE methods.
- Update (PUT): Modify an existing agreement’s metadata or participants.
- Delete (DELETE): Cancel or remove an agreement.
Acrobat Sign API Data Model Overview
It is important to understand the data models of the API we are going to integrate. Data model are essential for understanding data structure useful in storing and retrieving data from database. It helps in data integrity and consistency.
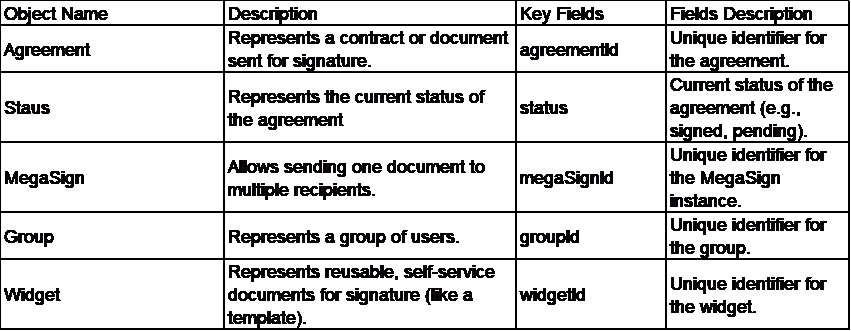
Advanced API Integration Features
The Adobe Acrobat Sign API provides advanced integration tools for integrating e-signature workflows into applications. Many enterprises such as Salesforce, Workday, Apttus, Ariba and more already collaborate and use Advanced API Integration Features that Adobe offers.
Acrobat Sign API Webhooks: Event-Driven Integrations
Webhooks enable service-to-service communication using a push model. They provide a more modern API solution by allowing real-time updates on agreement statuses. Set up webhooks to notify you when someone signs or cancels an agreement.
Building Custom Workflows and Templates with Acrobat Sign API
The Custom Workflow Designer lets you create tailored workflow templates for agreements. It helps you define the composition and signing processes to match your business needs. Workflow templates guide senders through the agreement creation process with custom instructions and fields. This makes the sending process easier.
User and Group Management
The User API assigns roles and manages permissions directly. The API allows for managing users, creating groups, and setting role-based access. Business and enterprise-level accounts get access to the group feature. Go to Accounts> Group. Here you can create, delete, modify and change group-level settings.
Automating Processes With Workflows
It streamlines tasks such as contract approvals, cutting down manual effort. Adobe offers many features for automating processes. These include a built-in visual design tool for task automation, document routing, and creating reusable templates for teams.
Bulk Data Operations
Bulk data operations ensure consistency by applying uniform changes across all items. They also increase efficiency and reduce the number of API calls. For example, you can use the Mega Sign feature to send agreements to multiple people, while providing a personalized experience for each signer.
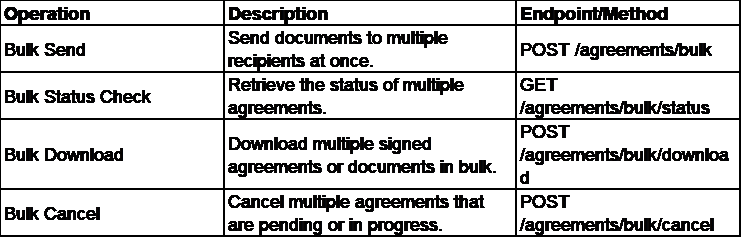
Security and Compliance
They are integral to the Acrobat Sign API, ensuring digital signatures meet legal standards. The API supports features like audit trails, encryption, and compliance with regulations such as eIDAS and ESIGN.
Integration With Knit
Knits Unified eSignature APIs offer many benefits for Acrobat Sign integrations. The Adobe Acrobat Sign API allows Knit users to automate workflows like onboarding, eliminating manual signatures and tracking. You just need to worry about integrating with one API Knit, and it takes care of rest. It eliminates complex download-print-sign-scan-email cycles by integrating directly with your existing systems.
Prerequisites for Integration
To integrate Adobe Acrobat Sign with Knit, you need to have:
- Adobe Acrobat Sign Account: Required for accessing the Acrobat Sign API.
- Knit Account: Ensure it supports API integrations.
- API Keys/Authentication Tokens: Obtain these from Adobe Sign and Knit.
- Developer Access: Ensure you have the necessary permissions.
Steps To Integrate Adobe Acrobat Sign With Knit
- Authenticate with Knit API: Use OAuth to establish a secure connection with Knit. Example: POST /oauth/token to get an access token.
- Sending documents for signing: Use the Acrobat Sign API's POST /agreements endpoint to send documents to employees for signing.
- Monitor Status: Retrieve the agreement status using the GET /agreements/{agreementId} endpoint.
- Example workflow: Knit provides a step-by-step guide for Sending Documents for Signature using Adobe Acrobat Sign.
Mapping Objects and Fields to Knit's Standard API
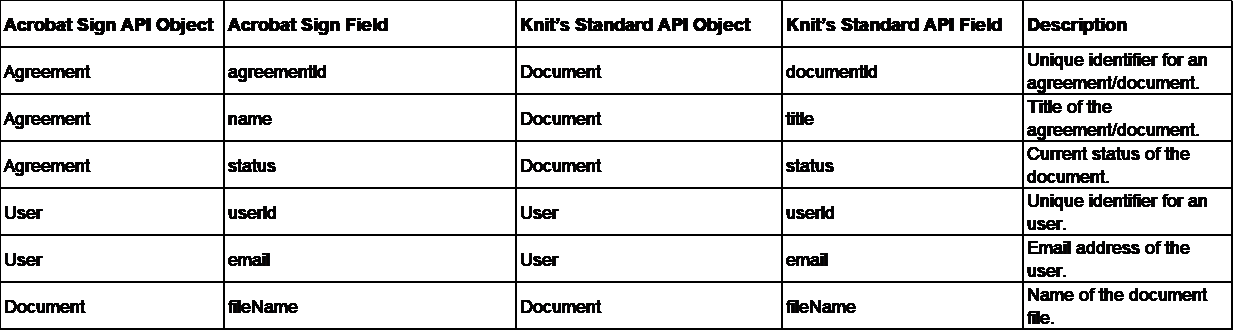
Testing and Validation
- Test: Send sample documents and check if they appear in Adobe Sign and Knit.
- Common Issues: Authentication errors, and incorrect API endpoint usage.
- Troubleshooting: Verify API keys, check response codes, and review integration logs.
Adobe API Integration Case Studies and Real-World Use Cases
Salesforce is a leading customer relationship management (CRM) platform. Salesforce's integration with Adobe Acrobat Sign is a great example of successful contract management and e-signature solutions.
Key benefits of the integration:
Salesforce users can directly access Adobe Acrobat Sign's features from within their CRM platform. Businesses within Salesforce can streamline contract creation, negotiation, and execution. You can create documents using ‘Document Builder’, gather e-signatures and store them securely to close business in no time. Speed up sales cycles by 90% when you use Acrobat Sign to gather e-signatures and automate workflows right within Salesforce.
Best Practices for Adobe Acrobat Sign API Integration
Adobe Acrobat Sign API Security Best Practices
Integrating the Adobe Acrobat Sign API effectively and securely requires developers to follow key practices to ensure data protection and seamless operation. Below are the best practices for secure integration:
- Protecting sensitive data: Use end-to-end encryption to secure document transfers and API interactions. Encrypt data both during transit and when stored using TLS 1.2 or higher. Implement OAuth 2.0 for secure, token-based authentication, so user credentials stay protected. Set precise user permissions in the Adobe Acrobat Sign API to limit access to sensitive documents.
- Secure API practices: Keep your APIs safe by rotating tokens regularly and storing them in environment variables—never in the codebase. Use OAuth 2.0 to reduce risks of credential-based attacks. Apply for least privilege access, ensuring each token has only the permissions it needs, lowering the chance of misuse.
- Monitoring and logging API usage: Monitor and log all API activity to catch issues early. Log details like timestamps, endpoints, and response times for troubleshooting and performance checks. Adobe Acrobat Sign API's monitoring tools help track usage and spot potential security threats or misuse easily.
Handling Adobe Acrobat Sign API Rate Limits and Errors
Effective error handling significantly improves your API integration. Here’s an overview of issues, error codes, and solutions:
Common Issues
- Authentication Failures – OAuth token expiration or misconfiguration can cause 401 errors. Ensure tokens are valid and permissions are correctly set.
- Incorrect API Endpoints – Using outdated or incorrect endpoints often leads to 404 errors. Always verify endpoints in the Adobe Sign API documentation.
Standard Error Codes:
- 400 (Bad Request): Invalid request format or missing parameters.
- 401 (Unauthorized): Token expired or invalid. Check authentication settings.
- 403 (Forbidden): Insufficient permissions. Ensure the app has the right scopes.
- 500 (Internal Server Error): Server issues. Retry the request or contact support.
- 429 (Too many requests): Exceeding API call limits triggers this error. Implement retry logic after the cooldown period when the system exceeds the rate limit.
Solutions
- Authentication Errors: Revalidate OAuth tokens by refreshing them periodically. Ensure client credentials are correctly configured.
- Incorrect API Usage: Cross-check API requests with the latest Adobe Sign API documentation. Review logs for potential mistakes.
- Rate Limits: Reduce the number of API requests or implement backoff strategies when nearing the rate limit.
Future Trends in Adobe Acrobat Sign API
With the increased demand for digital signatures, Adobe Acrobat Sign API is evolving to provide the best user experience. Here’s a look at future trends and what developers can expect.
Current Released Features in Adobe Acrobat Sign API
In the August 13, 2024 production deployment, Adobe Acrobat improved functionality and enhanced the user experience.
Improved Functionality
The Manage page has new links to the Power Automate Template Gallery, with the "In Progress" filter linking to Notification templates and the "Completed" filter linking to Archival templates.
You can access links by clicking the ellipsis next to filter labels or in the list of actions for selected agreements.
User Experience Changes
Changes such as a new post-signing page for unregistered recipients, a Change to the Send Code announcement for Phone Authentication and many others have been deployed.
Keeping Up With API Changes and Updates
Stay updated on AdobeSign API by regularly checking its documentation and release notes. Join developer communities and subscribe to newsletters for important updates.
Takeaway
The Knit Unified API simplifies the complex integration process. It manages all complex API operations, ensuring that your Adobe Acrobat Sign API setup remains efficient. This allows developers to focus on core tasks while staying future-proof.
By staying aware of these trends and leveraging tools like Knit, businesses can ensure long-term success with their Acrobat Sign API integration. To integrate the Acrobat Sign API with ease, you can Book a call with Knit for personalized guidance and make your integration future-ready today! To sign up for free, click here. To check the pricing, see our pricing page.
Adobe API FAQ: Common Questions and Answers
- Is the Adobe Sign API free?
Adobe Sign API offers free developer edition, with limited API usage.
- Are Adobe APIs free?
Adobe APIs are not entirely free. While some APIs, like the PDF Embed API, offer free tiers or usage limits, others require paid subscriptions or usage-based fees.
- Is Adobe request sign free?
Yes, Adobe Acrobat offers a free option for requesting signatures.
You can send documents for e-signing and track their progress without paying a fee. However, there are limitations to the free version, such as the number of documents you can send for signatures each month.