Jira is one of those tools that quietly powers the backbone of how teams work—whether you're NASA tracking space-bound bugs or a startup shipping sprints on Mondays. Over 300,000 companies use it to keep projects on track, and it’s not hard to see why.
This guide is meant to help you get started with Jira’s API—especially if you’re looking to automate tasks, sync systems, or just make your project workflows smoother. Whether you're exploring an integration for the first time or looking to go deeper with use cases, we’ve tried to keep things simple, practical, and relevant.
Understanding the Jira API
At its core, Jira is a powerful tool for tracking issues and managing projects. The Jira API takes that one step further—it opens up everything under the hood so your systems can talk to Jira automatically.
Think of it as giving your app the ability to create tickets, update statuses, pull reports, and tweak workflows—without anyone needing to click around. Whether you're building an integration from scratch or syncing data across tools, the API is how you do it.
It’s well-documented, RESTful, and gives you access to all the key stuff: issues, projects, boards, users, workflows—you name it.
Why Integrate with Jira?
Chances are, your customers are already using Jira to manage bugs, tasks, or product sprints. By integrating with it, you let them:
- Create and update tickets automatically
- Keep data in sync across tools
- Build dashboards that show real-time project health
It’s a win-win. Your users save time by avoiding duplicate work, and your app becomes a more valuable part of their workflow. Plus, once you set up the integration, you open the door to a ton of automation—like auto-updating statuses, triggering alerts, or even creating tasks based on events from your product.
Foundational Jira Concepts for Developers
Before you dive into the API calls, it's helpful to understand how Jira is structured. Here are some basics:
- Issues: These are the core units—bugs, tasks, features, etc.
- Projects: A collection of issues under one umbrella (e.g., a product or team).
- Boards: Visual tools for managing issues, especially in agile workflows.
- Workflows: The path an issue takes from "To Do" to "Done."
- Schemes: Settings that define permissions, notifications, and more.
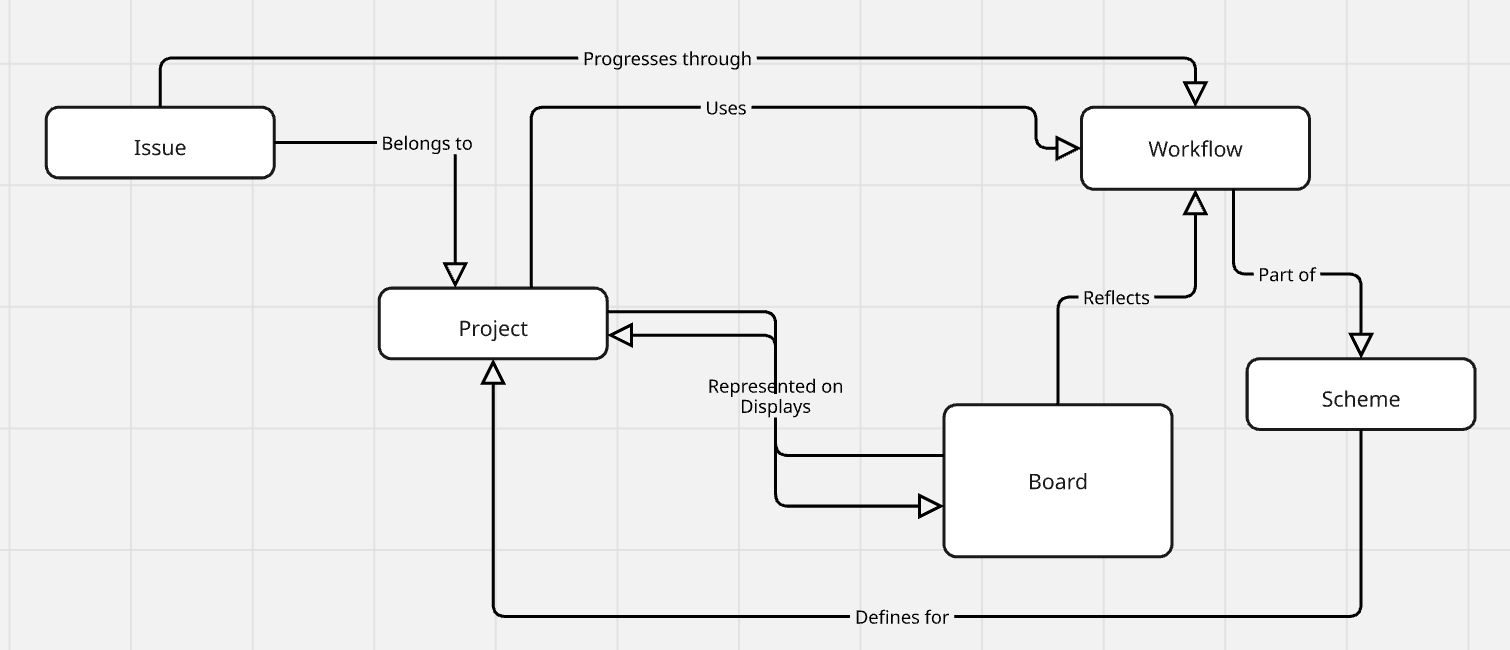
Each of these maps to specific API endpoints. Knowing how they relate helps you design cleaner, more effective integrations.
Preparing Your Development Space
1. Prerequisites
To start building with the Jira API, here’s what you’ll want to have set up:
- A language you’re comfortable with (Node.js, Python, Java, etc.)
- An HTTP client like Postman or curl for testing
- Version control (Git)
- Your favorite IDE or code editor
If you're using Jira Cloud, you're working with the latest API. If you're on Jira Server/Data Center, there might be a few quirks and legacy differences to account for.
2. Setting Up a Jira Test Environment (Highly Recommended)
Before you point anything at production, set up a test instance of Jira Cloud. It’s free to try and gives you a safe place to break things while you build.
You can:
- Spin up a trial via Atlassian’s website
- Use default settings to mimic real-world use
- Invite teammates for collaborative testing
Testing in a sandbox means fewer headaches down the line—especially when things go wrong (and they sometimes will).
Getting Started with the Jira API: The Basics
1. Navigating API Documentation
The official Jira API documentation is your best friend when starting an integration. It's hosted by Atlassian and offers granular details on endpoints, request/response bodies, and error messages. Use the interactive API explorer and bookmark sections such as Authentication, Issues, and Projects to make your development process efficient.
2. Authentication and Security
Jira supports several different ways to authenticate API requests. Let’s break them down quickly so you can choose what fits your setup.
A. Basic Authentication (Deprecated)
Basic authentication is now deprecated but may still be used for legacy systems. It consists of passing a username and password with every request. While easy, it does not have strong security features, hence the phasing out.
B. OAuth 1.0 (Deprecated)
OAuth 1.0a has been replaced by more secure protocols. It was previously used for authorization but is now phased out due to security concerns.
C. Utilizing API Tokens (Recommended)
For most modern Jira Cloud integrations, API tokens are your best bet. Here’s how you use them:
- Head to your Atlassian account and generate a token.
- Use basic auth with your email and the token instead of a password.
It’s simple, secure, and works well for most use cases.
D. OAuth 2.0 (3LO)
If your app needs to access Jira on behalf of users (with their permission), you’ll want to go with 3-legged OAuth. You’ll:
- Set up an app on Atlassian Developer Console
- Get the user’s consent via a browser flow
- Use the token you receive to make authenticated API calls
It’s a bit more work upfront, but it gives you scoped, permissioned access.
E. Forge & Connect Apps
If you're building apps *inside* the Atlassian ecosystem, you'll either use:
- OAuth 2.0 via Forge (for apps built on Atlassian’s new cloud dev platform)
- JWT (for legacy Connect apps)
Both offer deeper integrations and more control, but require additional setup.
3. Permissions & Rules
Whichever method you use, make sure:
- Your user/token has the right permissions (some endpoints need admin access)
- You handle errors gracefully (403s usually mean you’re missing access)
- You’re storing tokens securely (env vars, secret managers, etc.)
A lot of issues during integration come down to misconfigured auth—so double-check before you start debugging the code.
Managing Issues Using the Jira API
Once you're authenticated, one of the first things you’ll want to do is start interacting with Jira issues. Here’s how to handle the basics: create, read, update, delete (aka CRUD).
1. Creating Issues
To create a new issue, you’ll need to call the `POST /rest/api/3/issue` endpoint with a few required fields:
{
"fields": {
"project": { "key": "PROJ" },
"issuetype": { "name": "Bug" },
"summary": "Something’s broken!",
"description": "Details about the bug go here."
}
}
At a minimum, you need the project key, issue type, and summary. The rest—like description, labels, and custom fields—are optional but useful.
Make sure to log the responses so you can debug if anything fails. And yes, retry logic helps if you hit rate limits or flaky network issues.
2. Reading Issues
To fetch an issue, use a GET request:
GET /rest/api/3/issue/{issueIdOrKey}
You’ll get back a JSON object with all the juicy details: summary, description, status, assignee, comments, history, etc.
It’s pretty handy if you’re syncing with another system or building a custom dashboard.
3. Updating Issues
Need to update an issue’s status, add a comment, or change the priority? Use PUT for full updates or PATCH for partial ones.
A common use case is adding a comment:
{
"body": "Following up on this issue—any updates?"
}
Make sure to avoid overwriting fields unintentionally. Always double-check what you're sending in the payload.
4. Deleting Issues (Use with Caution!)
Deleting issues is irreversible. Only do it if you're absolutely sure—and always ensure your API token has the right permissions.
It’s best practice to:
Confirm the issue should be deleted (maybe with a soft-delete flag first)
Keep an audit trail somewhere. Handle deletion errors gracefully
5. Searching for Issues with JQL
Jira comes with a powerful query language called JQL (Jira Query Language) that lets you search for precise issues.
Want all open bugs assigned to a specific user? Or tasks due this week? JQL can help with that.
Example: project = PROJ AND status = "In Progress" AND assignee = currentUser()
When using the search API, don’t forget to paginate: GET /rest/api/3/search?jql=yourQuery&startAt=0&maxResults=50
This helps when you're dealing with hundreds (or thousands) of issues.
Managing Projects, Boards, and Workflows
1. Project Management via the API
The API also allows you to create and manage Jira projects. This is especially useful for automating new customer onboarding.
Use the `POST /rest/api/3/project` endpoint to create a new project, and pass in details like the project key, name, lead, and template.
You can also update project settings and connect them to workflows, issue type schemes, and permission schemes.
2. Agile Management: Boards and Sprints (Agile API)
If your customers use Jira for agile, you’ll want to work with boards and sprints.
Here’s what you can do with the API:
- Fetch boards (`GET /board`)
- Retrieve or create sprints
- Move issues between sprints
It helps sync sprint timelines or mirror status in an external dashboard.
3. Workflow Interactions via the API
Jira Workflows define how an issue moves through statuses. You can:
- Get available transitions (`GET /issue/{key}/transitions`)
- Perform a transition (`POST /issue/{key}/transitions`)
This lets you automate common flows like moving an issue to "In Review" after a pull request is merged.
Advanced Features and Webhooks
1. Leveraging Advanced Jira API Features
Jira’s API has some nice extras that help you build smarter, more responsive integrations.
2. Establishing Links Between Issues
You can link related issues (like blockers or duplicates) via the API. Handy for tracking dependencies or duplicate reports across teams.
Example:
{
"type": { "name": "Blocks" },
"inwardIssue": { "key": "PROJ-101" },
"outwardIssue": { "key": "PROJ-102" }
}
Always validate the link type you're using and make sure it fits your project config.
3. Establishing Links Between Issues
Need to upload logs, screenshots, or files? Use the attachments endpoint with a multipart/form-data request.
Just remember:
- Respect file size limits
- Watch out for unsupported types
- Secure file handling matters if you’re building a public-facing app
4. Implementing Event-Driven Integrations with Webhooks
Want your app to react instantly when something changes in Jira? Webhooks are the way to go.
You can subscribe to events like issue creation, status changes, or comments. When triggered, Jira sends a JSON payload to your endpoint.
Make sure to:
- Validate incoming payloads
- Log and retry failed deliveries
- Secure your endpoint (rate limits + auth)
5. Key Differences Between Jira Cloud and Jira Server
Understanding the differences between Jira Cloud and Jira Server is critical:
- Endpoints: Some endpoints differ in URL and available features.
- Version-Specific Features: Jira Cloud often has newer features not available in Server.
- Limitations: Be aware of any restrictions that may affect your integration.
Keep updated with the latest changes by monitoring Atlassian’s release notes and documentation.
Error Handling, Rate Limits, and Performance
Even with the best setup, things can (and will) go wrong. Here’s how to prepare for it.
1. Common Error Codes
Jira’s API gives back standard HTTP response codes. Some you’ll run into often:
- 400 Bad Request: Usually a missing field or invalid format. Double-check your payload.
- 401 Unauthorized: Your token might be missing or incorrect.
- 403 Forbidden: You’re authenticated, but don’t have permission to do the thing.
- 404 Not Found: The issue/project/resource doesn’t exist.
- 429 Too Many Requests: You hit a rate limit. Time to back off and retry later.
- 500 Internal Server Error: Something broke on Jira’s side. Try again with a delay.
Always log error responses with enough context (request, response body, endpoint) to debug quickly.
2. Handling Rate Limiting and API Throttling
Jira Cloud has built-in rate limiting to prevent abuse. It’s not always published in detail, but here’s how to handle it safely:
- Watch for `429` status codes
- Check headers like `X-RateLimit-Remaining` if available
- Use exponential backoff to retry: e.g., wait 1s, 2s, 4s, etc.
- Avoid sending bursts of traffic—spread out API calls where possible
If you’re building a high-throughput integration, test with realistic volumes and plan for throttling.
3. Strategies for Performance Enhancement
To make your integration fast and reliable:
- Use JQL wisely: Don’t pull every issue—fetch only what you need.
- Paginate properly: Jira caps results. Always check `startAt` and `maxResults`.
- Batch requests: If possible, group changes to reduce roundtrips.
- Cache where safe: For static metadata (like field definitions), caching improves speed.
- Async processing: Don’t make users wait—trigger long processes in the background.
These small tweaks go a long way in keeping your integration snappy and stable.
Logging, Debugging, and Testing
Getting visibility into your integration is just as important as writing the code. Here's how to keep things observable and testable.
1. Best Practices for Logging API Interactions
Solid logging = easier debugging. Here's what to keep in mind:
- Structure your logs: Use a consistent format (JSON works great for parsing).
- Log requests/responses: Especially when working with external APIs.
- Redact sensitive data: Mask things like API tokens before logging.
- Trace IDs: Use correlation IDs to trace a flow across systems.
If something breaks, good logs can save hours of head-scratching.
2. Approaches to Debugging Common Integration Issues
When you’re trying to figure out what’s going wrong:
- Postman: Great for testing individual API calls.
- curl: Quick and flexible for command-line requests.
- Request logs: Look at the exact payloads being sent/received.
- Jira UI: Check if what you're doing via API reflects in the frontend.
Also, if your app has logs tied to user sessions or sync jobs, make those searchable by ID.
3. Incorporating Automated Testing
Testing your Jira integration shouldn’t be an afterthought. It keeps things reliable and easy to update.
- Unit tests: Mock the API and validate your logic.
- Integration tests: Run tests against a test Jira instance.
- CI/CD: Plug your tests into your pipeline to catch regressions early.
The goal is to have confidence in every deploy—not to ship and pray.
Real-World Use Cases (Code Samples)
Let’s look at a few examples of what’s possible when you put it all together:
1. Auto-Creating Jira Tickets from Your App
Trigger issue creation when a bug or support request is reported:
curl --request POST \
--url 'https://your-domain.atlassian.net/rest/api/3/issue' \
--user 'email@example.com:<api_token>' \
--header 'Accept: application/json' \
--header 'Content-Type: application/json' \
--data '{
"fields": {
"project": { "key": "PROJ" },
"issuetype": { "name": "Bug" },
"summary": "Bug in production",
"description": "A detailed bug report goes here."
}
}'
2. Syncing Two Project Management Tools
Read issue data from Jira and sync it to another tool:
bash
curl -u email@example.com:API_TOKEN -X GET \ https://your-domain.atlassian.net/rest/api/3/issue/PROJ-123
Map fields like title, status, and priority, and push updates as needed.
3. Auto-Transitioning Overdue Tasks
Use a scheduled script to move overdue tasks to a "Stuck" column:
```python
import requests
import json
jira_domain = "https://your-domain.atlassian.net"
api_token = "API_TOKEN"
email = "email@example.com"
headers = {"Content-Type": "application/json"}
# Find overdue issues
jql = "project = PROJ AND due < now() AND status != 'Done'"
response = requests.get(f"{jira_domain}/rest/api/3/search",
headers=headers,
auth=(email, api_token),
params={"jql": jql})
for issue in response.json().get("issues", []):
issue_key = issue["key"]
payload = {"transition": {"id": "31"}} # Replace with correct transition ID
requests.post(f"{jira_domain}/rest/api/3/issue/{issue_key}/transitions",
headers=headers,
auth=(email, api_token),
data=json.dumps(payload))
```
Automations like this can help keep boards clean and accurate.
Keeping Your Jira Integration Secure
Security's key, so let's keep it simple:
1. Handle Secrets Right
Think of API keys like passwords.
- Env Vars: Store them there, not in your code.
- Encryption: For keepsies, encrypt them.
- Rotate: Change them regularly.
Secure secrets = less risk.
2. User Data - Be Smart
If you touch user data:
- Compliance: Know the rules (GDPR, etc.).
- Retention: Don't keep it forever.
- Access: Only those who need it see it.
Making Your Jira Integration Better
Quick tips to level up:
1. Client Libraries - Maybe?
Libraries (Java, Python, etc.) can help with the basics.
- Good for: Quick setup, common tasks.
- Less good for: Full control.
Your call is based on your needs.
2. CI/CD is Your Friend
Automate testing and deployment.
- Test often: Catch issues early.
- Deploy smoothly: Less manual work.
- Monitor: Keep an eye on things.
Reliable integration = happy you.
Conclusion and Final Checklist
If you’ve made it this far—nice work! You’ve got everything you need to build a powerful, reliable Jira integration. Whether you're syncing data, triggering workflows, or pulling reports, the Jira API opens up a ton of possibilities.
Here’s a quick checklist to recap:
✅ Before You Start
- Choose between Jira Cloud or Server/Data Center.
- Set up a sandbox/test environment.
- Pick your preferred auth method (API token, OAuth, etc).
🛠️ While Building
- Use the right endpoints for issues, projects, boards, and workflows.
- Test API calls in Postman or curl.
- Log requests and responses (without leaking sensitive data).
- Handle common errors and rate limits
- Optimize with pagination, batching, and caching
🚀 Before Shipping
- Write automated tests (unit + integration).
- Monitor logs for failures or edge cases.
- Document your field mappings and logic.
- Validate your webhook setup if used.
Keep Exploring
Jira is constantly evolving, and so are the use cases around it. If you want to go further:
- Follow [Atlassian’s Developer Changelog]
- Explore the [Jira API Docs]
- Join the [Atlassian Developer Community]
And if you're building on top of Knit, we’re always here to help.
Drop us an email at hello@getknit.dev if you run into a use case that isn’t covered.
Happy building! 🙌